Intercepting HTTP requests with a DelegatingHandler
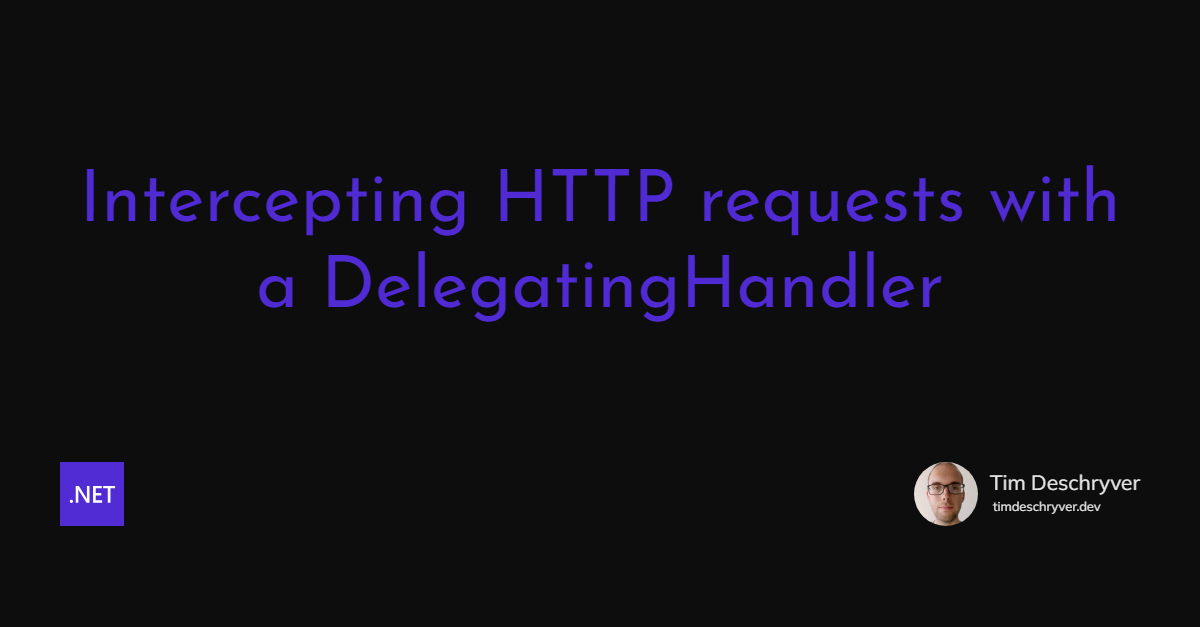
Intercepting HTTP requests is a way to extract common infrastructure logic that you don't want to have in your application code.
It allows you to perform some logic before the request is made, or after the response is received.
In ASP.NET an interceptor can be implemented by using a DelegatingHandler
.
A use case for an interceptor is adding a header, e.g. the Authorization header, to the HTTP request.
When this is a static value, configuring a default header during the setup of an HttpClient
is the better and easier choice, but when the authorization's header value needs to be dynamic then this isn't an option.
Another use case might be logging purposes, or handling errors that might occur.
In this blog post, I'll be using the use case of adding a request header to zoom into the implementation.
As a general best practice we don't want to insert (common) technical behavior within the application's logic. Invoking HTTP requests is no different. We don't want to pollute how HTTP requests are made with too many details. We also need to watch out that this logic isn't shattered all over the place. If we're not attentive we might end up with different versions of its implementation, and when the requirements should change in the future we need to find and modify the new behavior in multiple locations. This is error-prone and time-consuming.
DelegatingHandler
s is a solution to this problem by providing a central point where that logic can exist.
Common behavior can be written once, and is automatically applied to all made HTTP requests (for a given HTTP client).
Because the common logic is extracted it also becomes easier to write tests and to change its behavior in the future when requirements are changed.
Implementing a DelegatingHandler
link
Let's take a look at an example implementation to add a header to the requests using a DelegatingHandler
.
If the class constructor's syntax is a bit funky, be sure to check out the Primary Constructor syntax, which is added in C# 12.
Configuration link
To make use of the delegating handler, add it within the DI container and register the handler to a specific HTTP client using AddHttpMessageHandler
.
After these changes requests that are invoked via the ExternalClient
will pass through the ExternalServiceAuthenticationHeaderHttpInterceptor
delegating handler.
Conclusion link
In this blog post, I've shown how to implement a DelegatingHandler
.
A DelegatingHandler
allows us to intercept an HTTP request to perform common behavior before a request is fired or after a response is received.
This helps to remove redundant code, keeping the application's logic clean and compact. The dev team doesn't need to pay attention to this detail while reading existing code, nor does the team need to be reminded of this behavior while writing new functionality that interacts with the service.
For more information about DelegatingHandler
s, check out the official documentation.
Outgoing links
Feel free to update this blog post on GitHub, thanks in advance!
Join My Newsletter (WIP)
Join my weekly newsletter to receive my latest blog posts and bits, directly in your inbox.
Support me
I appreciate it if you would support me if have you enjoyed this post and found it useful, thank you in advance.