Good testing practices with 🦔 Angular Testing Library
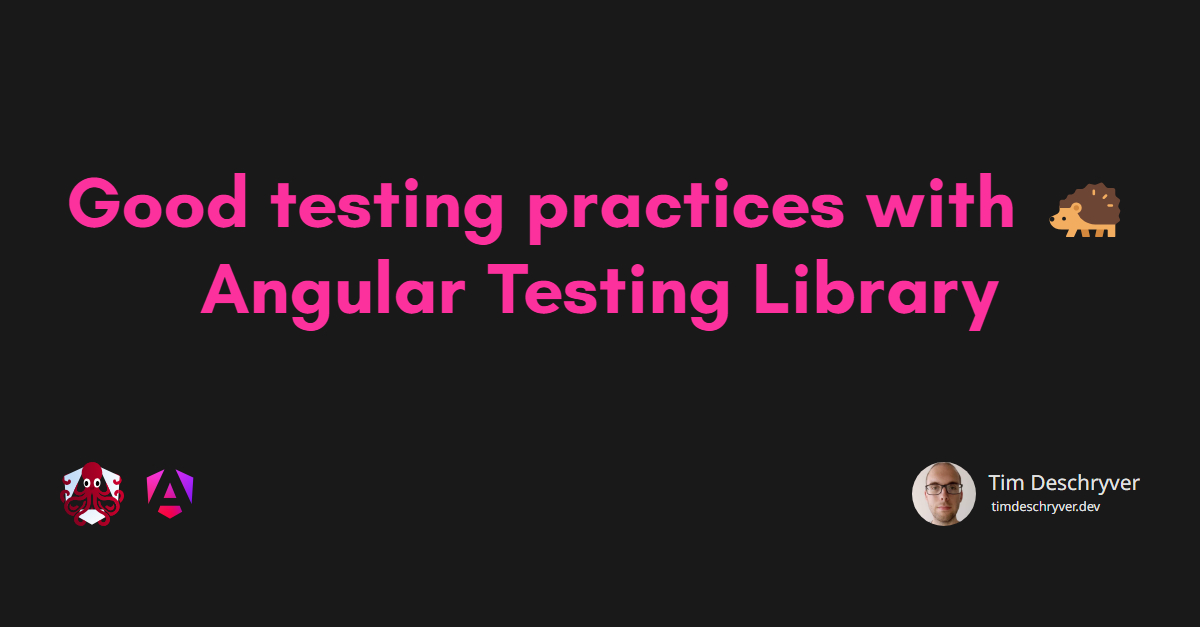
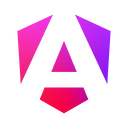
Angular Testing Library
provides utility functions to interact with Angular components, in the same way as a user would. This brings more maintainability to our tests, gives us more confidence that our component does what it's supposed to do, and it improves the accessibility which is better for our users. All these benefits, plus you'll see that it's fun to write tests in this way.
The Angular Testing Library
link
Angular Testing Library is a part of the @testing-library family with 🦑 DOM Testing Library in the center of the family. We're encouraging good testing practices across multiple frameworks and libraries by bringing a similar API to the table. These tests can be written in the test runner of your liking.
We encourage:
- maintainable tests: we do not want to test implementation details
- confidence in our components: you interact with your components the same way as your end-users
- accessibility: we want inclusive components
format_quoteThe more your tests resemble the way your software is used, the more confidence they can give you.
Getting Started link
To get started, the first step is to install @testing-library/angular
, after that we're good to go.
In this post, we'll take an introduction by writing tests for a feedback form, starting very simple and keep building on top of it.
The form we'll put under test has a required name field, a required rating field that must be between 0 and 10, a summary field, and a select box to select a t-shirt size. A form wouldn't be a form without a submit button, so let's add that too.
The code of the forms looks as follows.
Our first test link
To be able to test the feedback component we must be able to render the component, we can do this by using the render
function.
The render
function takes the component under test as the first argument and has an optional second argument for more options see RenderOptions
, which we'll be covering soon.
format_quoteIt doesn't have to be more than this as setup to test a simple component
In this case, it throws an exception because we're making use of reactive forms and some of the Angular Material components.
To solve this we must provide both of the missing modules. To provide these modules we use the imports
property on the RenderOptions
, similar to how TestBed.configureTestingModule
works.
Now, this test works.
Queries link
The render
function returns a RenderResult
object which contains utility functions to test the component.
You'll notice that we test components in a similar way just as an an end-user would do.
We don't test implementation details but Angular Testing Library
gives us an API to test the component from the outside, via the component DOM nodes.
To verify the nodes an end-user sees we use queries, these are available on the rendered component.
format_quoteA query looks for the given text (as a
string
orRegExp
) in the component, which is the first argument of the query. The optional second argument is TextMatch.
In our test, to verify that the form is rendered with the correct title we can use the getByText
query.
To use the query, we must import the screen
object first.
You can think of screen
, as the view that a end-user sees, it contains DOM of the page.
In the above snippet, you don't see an assertion. This is because the getBy
and getAllBy
queries throw an error when the query isn't able to find the given text in the document. If we don't want Angular Testing Library to throw an error we can use the queryBy
and queryAllBy
queries instead, these queries return null
if the element(s) can't be found.
For async code, it's also possible to wait for a moment until the element becomes visible or until a timeout occurs.
If you want to test async code, the queries you're looking for are findByText
and findAllByText
.
Before every check if the element is visible, Angular Testing Library will trigger a change detection cycle.
Setting @Input()
and @Output()
properties
link
With the component rendered, the next step is to provide the needed @Input()
and @Output()
properties.
To assign these properties, we can use componentProperties
from the RenderOptions
.
In the case of the feedback component, we assign shirtSizes
to a collection of t-shirt sizes and we assign submitForm
to be a spy. Later on, we can use this spy to the verify the form submission.
Another way to do this, is to use the template syntax. This wraps the component into a host component.
With this step, the component is ready to start writing tests.
Events link
So far we've seen how we can assert our rendered components with query functions, but we also need a way to interact with our components. We can do this by firing events. Just like the query functions, these events are also available on the rendered component.
format_quoteFor the full list of supported events you can take a look at the source code. This post only covers the ones it needs to test the feedback component, but all of the events have a comparable API.
The first argument of an event is always the targeted DOM node, the optional second parameter is to provide extra information for the event. An example is which mouse button was pressed, or the text of an input event.
Good to know: an event will run a change detection cycle by calling detectChanges()
after the event is fired.
Clicking on elements link
To click on an element, we use the fireEvent.click
method.
Because we're able to click on the submit button now, we can also verify that the form has not been submitted because it's currently invalid.
We can use the second parameter (the options, which are the equivalent to the options of a JavaScript click) to fire a right click.
Filling in input fields link
To make the form valid, we must be able to fill in the fields. We can use this by using various events.
Just like before, we can get our form fields by using queries. This time we get the form fields by their corresponding label, this has the benefit that we're creating accessible forms.
format_quoteThe
getByLabelText
andqueryByLabelText
are also looking ataria-labels
to find an element
In the above form example, we notice that there are two different API's to fill in the input field.
The first one is via the input
method and the second one with the type
method from userEvent.
The difference between both API's is that input
just fires the JavaScript input
event to set the form value.
Whereas type
(from userEvent
) also replicates the same events an end-user would trigger while interacting with our form to fill in the form field. This means that the input field also receive other events like keydown
and keyup
. Besides that, that API from the userEvent
methods is also easier to work with. Because of these two reasons, this is the preferred way to interact with the component in your tests.
Invalid controls link
So far we have a working feedback component, but how could we test the validation messages?
We already have seen how we can verify our rendered component with queries
and we've also seen how we interact with the component by firing events, this means we got all the tools in our toolbelt to test invalid controls in the feedback form.
If we blank out an input field, we should see a validation message. This looks as follows.
Because a query returns the DOM node, we can use the DOM node to verify the control is valid or invalid.
Working with containers and components link
The current test covers our feedback component, which is just a component. For some scenarios this might be a good thing to do, but more often I'm of the opinion that these tests add no value. What I like to do is to test container components. Because a container consists of one or more components, these components will also be tested during the test of the container. Otherwise you will usually end with the same test twice, and with a double of the maintaining work.
To keep it simple, we simply wrap the form component in a container. The container has a service injected to provide the t-shirt sizes, and the service also has a submit function.
In the test for the FeedbackContainer
, we now have to declare FeedbackComponent
and provide a stubbed version of FeedbackService
.
To do this, we can use a similar API as TestBed.configureTestingModule
and use the declarations
and providers
properties on the RenderOptions
.
Besides the setup our test looks the same. In the test below, I choose to write the test in a more compact way which I find to come in handy for bigger forms.
A couple of tips for writing tests link
For End to End testing with Cypress use 🐅 Cypress Testing Library
link
Because it's part of @testing-library
, you can use a similar API while using Cypress.
This library exports the same utility functions from DOM Testing Library
as Cypress commands.
More info and examples can be found at @testing-library/cypress.
Use @testing-library/jest-dom
to make assertions human readable
link
This is only applicable if you're using Jest as your test runner.
This library has useful utility Jest matchers, for example toBeValid()
, toBeVisible()
, toHaveFormValues()
, and more.
More info and examples can be found at @testing-library/jest-dom.
Prefer one test over multiple tests link
As you have noticed in the snippets used in this article, they are all part of one single test. This goes against a popular principle that you should only have one assert for a test. I usually have one arrange and multiple acts and asserts in one test.
format_quote"Think of a test case workflow for a manual tester and try to make each of your test cases include all parts to that workflow. This often results in multiple actions and assertions which is fine."
Read more about this practice can be found at Write fewer, longer tests by Kent C. Dodds.
Don't use beforeEach
link
Using beforeEach
might be useful for certain test cases, but in most cases, I prefer to use a simple setup
function.
I find it more readable, plus it's more flexible if you want to use a different setup in various tests, for example:
Example code link
The code from this article is available on GitHub.
Once you know queries
and how to fire events, you're all set to test your components.
The only difference between the test case in this post and other test cases lies on how to set up the component with the render
function, you can find more examples in the Angular Testing Library
repository.
- Single component
- Nested component
@Input()
and@Output()
- Form
- Form with Angular Material
- Component with a provider
- With NgRx
- With NgRx
MockStore
- Testing a directive
- Router navigation
- Injection Token as a dependency
- Create an issue if what you're looking for is not in this list
Incoming links
Feel free to update this blog post on GitHub, thanks in advance!
Join My Newsletter (WIP)
Join my weekly newsletter to receive my latest blog posts and bits, directly in your inbox.
Support me
I appreciate it if you would support me if have you enjoyed this post and found it useful, thank you in advance.