Be proactive when you join an Angular project
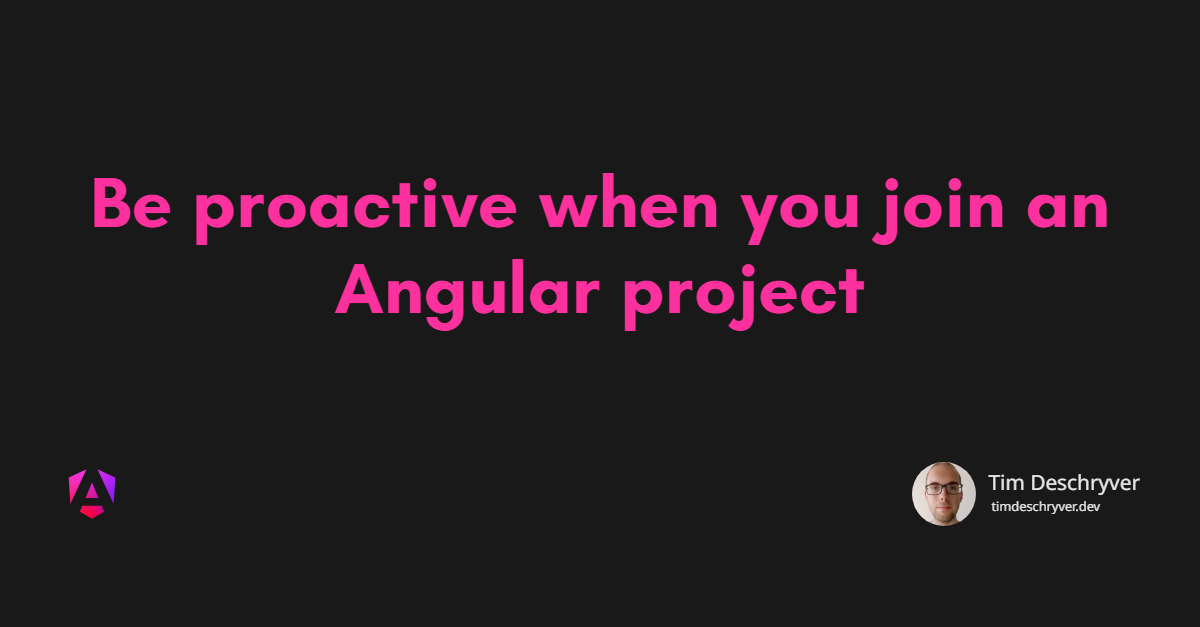
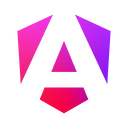
As developers, we're always using analogies to explain our work to non-developers. We do this to give an idea of what it's like without using too much of our technical jargon. In this intro, I'm doing the same.
Working on a project is a lot like gardening. You start to plant seeds and watch them slowly grow into a beautiful, colorful, garden, and a home to some animals. When you start out and everything is new, you give your new garden a lot of attention, but then you realize that it requires a consistent commitment to properly maintain the garden. If you want to do it right, you have to give it the required time, otherwise it transforms into a wilderness with dead flowers. Because the garden keeps on growing it becomes harder and harder to give each area the desired time. Sadly, you also need to attain other chores that you don't like as much as maintaining your garden. This is all taking away valuable time the garden needs. Eventually, the garden slowly loses its beauty and so does your motivation.
Starting a new project is a lot like the above-described garden. The project starts off with good intentions, but eventually some trade-offs need to be made along the way due to several factors. Doing your best with the current information, deadlines that had to be reached, members that leave the team, evolving technology, and the lack of experience are all reasons that decide the quality of the codebase. When it becomes worse it also has an impact on the future development, at its worst-case, it also lowers the team mood and team morale. Making a new change requires more energy than it used to.
When you join a team it usually takes a while to be brought up-to-speed and to start being or feeling productive. When you hear your team members complain about the current state of the codebase, that it got out of control, that it could need a polishment, or when you notice it yourself, there's a chance for you to make a positive impact by being proactive.
Giving a codebase the required, but long over-due focus is a win-win situation for you and the team. You get an introduction to the codebase, and the whole team wins because you improve the state of the application. Because, let's be honest, no one else wants to do it at this point in time. It's also a perfect opportunity to get to know your team members because what you're about to do is going to result in questions on both sides.
Let's take a look at what you can do now to clean up the codebase by bringing it up to date. Let's start gardening!
Tune up the strictness link
TypeScript link
This is probably the most impactful item on your to-do list, but also the most time-consuming.
With strictness enabled it results in silly mistakes being caught at compile time, and thus you create a safer environment.
For example, it can detect an unsafe operation on an object that could possibly be null
.
To enable "strict mode" in your application, open the tsconfig.json
file and set the compilerOptions.strict
property to true
.
strict
is a superset containing multiple strict options. Besides the strict
flag, you can also enable more properties that result in a safer environment, for example, noImplicitOverride
, noPropertyAccessFromIndexSignature
, noImplicitReturns
, and noFallthroughCasesInSwitch
, which are enabled by default in a new Angular 13 project.
Angular templates link
Besides the TypeScript strict mode, Angular also provides a strictTemplates
option.
The option can be compared to the strict
option, but for the HTML templates.
For example, it warns you when you pass a method the wrong type, e.g. a string instead of a number.
The strictTemplates
option can be enabled by setting the angularCompilerOptions.strictTemplates
to true
in the tsconfig.json
file.
Enable strict mode link
The first time when you enable one or both strict options you are probably going to see some errors when you try to run )and build) the application. These need to be addressed first, before the application can run again.
See the TypeScript docs and the Angular docs for more info.
Betterer link
Refactoring all the errors at once is the best scenario, but don't worry when there are too many of them. Luckily, Betterer provides a solution to incrementally improve the state of the codebase.
By using Betterer, you're not forced to fix all the errors in one go, but the development process can continue. This means that you don't have to "waste" time before you can run the application. With Betterer you can take your time to take care of the errors one by one, without the addition of new errors, and this can be a team effort.
To add Betterer run the init command:
Then, you can remove the strict
option from the tsconfig.json
file (the one we added before) and move them to a Betterer test inside the .betterer.ts
file.
Before you run the Betterer command, also add the --strict
flag to the added betterer script in the package.json
file, making it harder to cheat with the test results.
Now, you're ready to run Betterer for the first time, which gives you the following result.
As you can see, the Betterer command checks for violations that are configured in the test file.
In this case, with the TypeScript strictness enabled.
What you can't see, is that it stores the results in a separate .betterer.results
file.
The next time that the command is run, Betterer compares the two results and throws an error when the result has gotten worse.
Great! You can now detect new violations and prevent them from being committed (more on this later).
When you've positively made improvements, Betterer lets you commit the changes, and it updates its results file.
Multiple tests can be added to the .betterer.ts
file, for example, we can also include a test for the Angular strict templates option.
Update Angular link
Another item on your to-do list is to verify that the project is running on the latest version of Angular. If you notice that this isn't the case you can try to update Angular. Most of the time, it only takes a few minutes, up to an hour, for it to complete. When you notice that this isn't the case, you can abort the upgrade progress and document what went well and what didn't, this is valuable information to schedule the upgrade. Also, if you notice that the latest version of Angular is just released a few weeks before, ask your colleagues if it's OK to upgrade Angular because there might be a policy that restricts this.
Updating Angular's dependencies isn't difficult, and there's an official Angular Update Guide that lays down the details and gives a step-by-step update path.
To check if a dependency can be updated, run the ng update
command.
If the project isn't using the latest version, you'll see an output like the one below.
Next, pass the desired libraries as an input argument to the ng update
command and let the Angular CLI do its magic.
format_quoteRead about how you can include your own libraries to the update command in ng update: the setup
ESLint link
In the early years Angular relied on TSLint to statically analyze your code to quickly find problems (also known as a linter) in an Angular project. In 2019-2020, TSLint became deprecated and was ported over to ESLint as typescript-eslint.
Because TSLint was included with the creation of a new Angular project, a lot of older Angular projects still depend on TSLint. This gives us another item on our to-do list, the migration from TSLint to ESLint.
For Angular projects, there's the angular-eslint ESLint plugin, which is the ESLint equivalent of codelyzer
.
Luckily, the angular-eslint
team has put a lot of effort into an automatic migration to offer us a smooth transition going from TSLint to ESLint. To upgrade your project to ESLint run the following commands.
The script ports over the TSLint rules to ESLint rules and tries to find the ESLint equivalents to your installed TSLint plugins. While you're installing and configuring ESLint, I recommend you to also add the RxJS ESLint plugin and if your project is using NgRx, there's also the NgRx ESLint Plugin.
Besides being useful (it can detect common mistakes), linters also includes fixers for some deprecations and best practices.
For a simple project, this results in the following ESLint config.
Before pushing these changes, let's also run ESLint against your whole codebase and let it fix violations automatically by using the --fix
flag.
This already solves a lot of issues, but for other issues you need to manually rewrite the code that causes the violation. To see all the ESLint errors and warnings, run the following command.
Here again, you can resort to Betterer if there are too many errors to fix at once by using the built-in Betterer ESLint Test.
Prettier link
Because everyone has a unique writing (and format) style, it sometimes makes it harder to review a change. By enforcing a team style you make sure that a change is isolated to just the task and nothing more. This practice makes it easier to review changes.
To enforce the writing style you can use Prettier, an opinionated code formatter.
To add prettier to your project, run the next command.
Then, create a prettier.config.js
config file and configure the options to your desires, for example:
I also encourage you to immediately run prettier on the whole project. Otherwise, a small change to a file may result in a lot of formatting changes in that same file, making it harder to review the isolated change.
To format your whole codebase at once, run the following command.
Prettier with ESLint link
You can end up with conflicts because both ESLint and Prettier can change your code. One can override the other, resulting in a different output. In the worst case, one rule might conflict and report warning(s) while the code looks good for the other.
To solve this, install the Prettier ESLint Plugin eslint-plugin-prettier
. Next, add the prettier plugin to the ESLint settings.
For using the plugin correctly with the Angular ESLint Plugin, you can take a look at the documentation.
Unifying libraries link
There might be some inconsistencies on a project where different developers have been working on. For example, different libraries that are used to do the same thing, e.g. icon libraries, utility libraries,...
For new developers that join the team, this makes it harder to follow the best practices and to keep the design consistent. It's up to you to detect these libraries and unify them into one library. Better, you can also document how something is best done in your project.
An additional benefit is that the bundle size shrinks.
Writing Tests link
On a project that doesn't have tests, there might be a fear of regression to touch existing parts of the application. To give you a layer of security, I find that end-to-end tests offer a lot of value. It also gives you a chance to go through the application and get acquainted with the domain.
A simple happy-path test to a critical part of the application is good enough to start with. While this provides direct value, it also acts as a good foundation that can be built upon.
To write the end-to-end test, I'm currently using Playwright. One of the key essentials is that it has a test generator command where you can just click through your application, and the generator writes the test case for you. It can just be that simple.
In future blog posts, I probably go into more details about why I like and use Playwright and how to set it up in an Angular project.
Git hooks link
The above-mentioned tools and rules are a great way to improve and maintain the state of the project, but it isn't a one-time thing, and we're also lacking a way to enforce them to the whole team and future developers.
Simply mentioning and documenting what you've done, and asking the team to pay attention to keep the application in a better shape isn't good enough. While the thought of it makes the team happy, in practice, these good intentions tend to evaporate quickly.
To force the team to follow these rules, you need to introduce git hooks. A hook is executed before (pre) or after (post) running a git command.
Usually you write a hook that runs before the git command is executed.
Two popular hooks are the pre-commit
and pre-push
hook to prevent "falsy" code is committed or pushed to a branch.
In the example below a pre-commit
file is created in the .githooks
folder, in which you implement the pre-commit
hook.
The implementation of the hook can run your npm scripts, in this case we want to run Betterer with the precommit
option, and we want to run lint-staged.
To register the git hook, add the prepare
lifecycle hook to the scripts of the package.json
file. When a team member runs the npm install
command, the prepare
hook is executed and the git hook is registered.
The lint-staged is a library (yes, another one) that helps us to keep the application in better shape. It makes it easy to run commands to staged git files before these are committed. In other words, a touched filed is automatically formatted (important if someone's IDE doesn't run prettier) and is checked against ESLint and strictness violations. This makes sure that code is always formatted and ensures that there are no violations against strict compilers and ESLint rules. You could also always run the commands to the whole project, but this could take up some time, using lint-staged makes sure that almost no time is lost.
To install lint-staged, run the following command.
To configure lint-staged, create a lint-staged.config.js
file and add the prettier
and eslint
commands.
Detect and remove unused objects link
A project that's been under development has probably a few objects (interfaces, methods, ...) that aren't used anymore. This is poluting the codebase and causes confusion.
You can detect these object with ts-prune. When you run the command below, you get a list unused objects.
Take a look at the output and act accordingly by removing unused objects, resulting in a clean code base.
NX link
Instead of manually upgrading the project to use the latest versions of the above mentioned tools and libraries, take a look at NX.
NX offers a rich eco-system and provides automatic upgrade paths.
Conclusion link
Joining a new team is always exciting and you never know in which shape you'll encounter the application. To start things off on a positive note there are probably some chores that you can pick up that no one else feels like doing.
By listening to the complaints and by taking a closer look at the codebase, I'm sure that you can find things that need to be improved. Sadly, no codebase is perfect. In these cases, I say "be proactive" and get to work.
This benefits you because you can get to know the application and your team members better while immediately making a good impact. It also benefits the whole team because a codebase that is well maintained leads to a better mood and motivates the team to continually improve. Because the morale is better, productivity also increases, making the managers happy.
Enjoy and take care of your clean workspace!
Outgoing links
Feel free to update this blog post on GitHub, thanks in advance!
Join My Newsletter (WIP)
Join my weekly newsletter to receive my latest blog posts and bits, directly in your inbox.
Support me
I appreciate it if you would support me if have you enjoyed this post and found it useful, thank you in advance.