NgRx creator functions 101
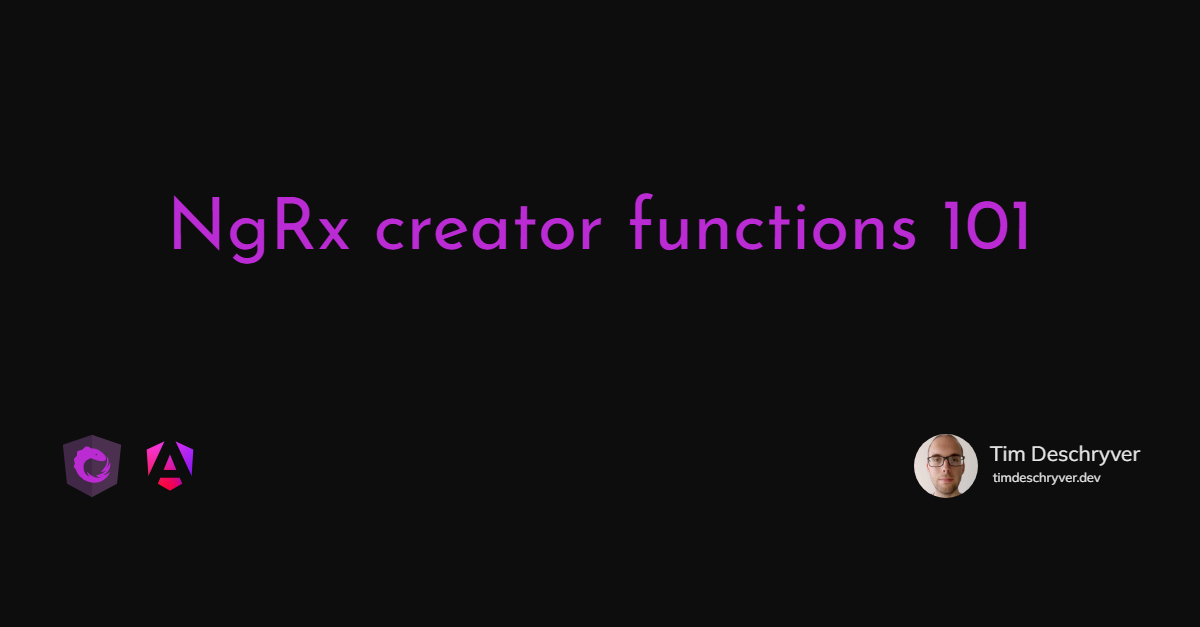
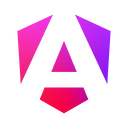
The createAction
creator function opened opportunities in the NgRx world. With it came two other creator functions, createReducer
and createEffect
. Let's take a look at what's so special about it and why it's important.
format_quoteThe creator functions were introduced in NgRx v8
Action link
In previous versions of NgRx, defining a new action took 3 steps.
The first step was to create the action type, usually as a string or as an enum value.
Then an action creator was defined as a class, implementing from the Action
interface from NgRx.
Lastly, the action needed to be added to a grouping of actions, also known as a union type.
The action types are used in reducers and effects. A type plays an important role because it's based on this type that reducers and effects filter out actions that they are interested in.
Via the action class, an action instance can be created. These instances are dispatched to the NgRx store.
To provide type-safety, the union type (and classes) are used to type the incoming actions.
createAction
link
With the added createAction
method, we achieve the same result with a single step.
The action creator is defined by invoking the createAction
function with the action's type and an optional payload.
The return type of the createAction
function is the ActionCreator
type. This is not just an action creator function, but it also has a type
property attached to the function.
This will be important for the createReducer
and createEffect
creator functions.
createAction
has three different styles:
- without a payload
- with a
props
payload
- with a function
Internally, the Object.defineProperty
method is used to create the type
property on the action creator.
Depending on how the action is configured, createAction
will create a function:
- without a payload, return a parameterless function and return the type
- with
props
, return a function that has the action's payload parameter and return payload with the added type - with function, return a function that has the function's parameter as parameter; the function is invoked and the output is returned with the added type
Reducer link
NgRx wouldn't be as powerful as it is, without having (the possibility to create) type-safe reducers.
The ActionTypes
enum and ActionsUnion
union type are used to make a reducer typed.
To have a type-safe reducer, we have to add the types a little bit manually.
This is done by typing the incoming action's type as the ActionsUnion
.
If you are handling an action from another feature, it's possible to add the specific type by creating an "inline union type".
By creating a switch
statement on the incoming action's type, TypeScript can infer the action's type within a case clause statement.
To use the action creators in a reducer, we have to make two changes.
First, we have to use the ActionCreator
's type property in the switch statement instead of the enum.
Secondly, to not lose the type-safety we have to type the incoming action.
Just like before, we have to create a union type.
But we can't simply create a union of the ActionCreator
s, but we need to access the actual action. Therefore we use the ReturnType
utility type of TypeScript, this will get us the return value of the action creator. In other words this gets us the actual action.
createReducer
link
To fully make use of the ActionCreator
's power, we can take it up another level. With the createReducer
function, creating a type-safe reducer becomes easier.
Instead of a good old switch statement, createReducer
uses on
functions to handle the actions and reduce a new state.
The on
function expects at least one ActionCreator
, and the last argument is an action reducer. An action reducer can be compared to a normal reducer function. It has the current state and the action as parameters, and it returns a new state.
Because on
works with ActionCreator
s, NgRx can infer the action and you have type-safety out of the box. Neat!
Internally, it uses the type
property on the ActionReducer
to know which on
reducers should be invoked.
There are two differences with the createReducer
function, compared to a reducer function.
- it doesn't need a default case to return the current state; if the action type can't be found in an
on
function within the reducer,createReducer
returns the current state - a switch statement can only react once to an action type; with the
on
function it's possible to react to the same action more than once in a single reducer
Let's take a look at the internal workings of the on
and createReducer
functions.
The on
function plucks all the types of the given ActionCreator
s.
It returns the list of action types and the action reducer function.
The createReducer
uses a Map
to know which on
function it should invoke. The Map
uses the action types as key, and has the action reducer function as the value for the coupled reducer to the action type.
To populate the map, it loops over all on
functions, and next it loops over all the action types. If the action type is already added to the map, it wraps the existing reducer with new reducer. Wrapping the reducer function ensures the second reducer receives the updated state. This can be done because all of the reducers look the same (they all have a state and an action as arguments, and they all return state), If it's the first time that the action type is encountered, it will simply be added to the map.
The createReducer
function returns a reducer function, which is invoked when an action is dispatched.
When the reducer function gets invoked, it uses the incoming action's type to find the to be invoked reducer based on the populated map. If the reducer function does not handle the incoming action, it will simply return the current state.
Effect link
To create a NgRx Effect in previous versions, the Effect is decorated with the @Effect()
decorator.
Here, again, we have to manually type the Effect, or the Actions
, to have the type-safety in place.
In the Effect, it's the ofType
operator that adds the type to the action.
Just like the reducer, the operator uses the action's type to know if it should handle the action and to provide type-safety throughout the next operators.
The first option to achieve a type-safe Effect is to add a generic to the ofType
operator. The generic is the action's class, and we give it the action's type (the enum value) as parameter.
The second option (introduced NgRx 7) to offer type-safety in the Effect, is to provide a generic on the Actions
class. Similar to the reducers, we can use the union type for it.
Because of this change, it also makes the ofType
operator smarter. It isn't needed anymore to type every single ofType
operator.
With the added ActionCreator
the ofType
operator is smart enough to infer the action's type.
The internal code of the ofType
operator looks as follows.
As you can see, it's the attached type
property on the ActionCreator
that makes this check possible.
createEffect
link
At first sight, the createEffect
function doesn't offer anything special, we already know that it's the combination of the ActionCreator
and the ofType
operator that does the trick. So what does createEffect
give us?
A downside of the @Effect
decorator is that its return type can not be checked at compile time. It's possible to not return an action, and when this happens it results in a runtime error (because the store expects an action to have a type
property).
This what the createEffect
function solves. It adds a check at compile time to protect ourselves from making this mistake.
Instead of using the @Effect
decorator, wrap the Effect's logic inside a createEffect
function.
The internal workings of an Effect are still the same, so I will not cover the code in this article. You can take a look at the source code if you're interested.
Tips link
A DRY reducer link
Inside a switch statement, you could group state clauses so it executed the same statement for different actions:
With the createReducer
, the on
function accepts more than one action creator as parameter to offer the same possibilities as a switch statement:
A composable reducer link
The createReducer
opens up the ability to compose a reducer because it can handle the same action multiple times. This isn't possible with a switch statement.
For a use case see the original issue that led to this change, by Siyang Kern.
Using an Action inside createReducer
link
For Action
s that are not converted to the new ActionCreator
's syntax, but need to be used inside a createReducer
function, a wrapper has to be written.
The wrapper will not be used in the code to dispatch the action, but will be used to comply with the on
signature.
For actions without a payload this can be done as:
Or an action with a payload:
Internally, NgRx used this approach for the router actions and effect actions. See the Pull Requests ROOT_EFFECTS_INIT actions as ActionCreators - by Sam Lin and add action creator for root router actions - by Jeffrey Bosch.
Migrating to createEffect
link
There's a schematic to convert all the @Effect
decorators to the createEffect
function, run the following command to run the schematic:
The NgRx Schematics has to be installed to run the command.
More info about the typings link
Alex Okrushko gave a talk Magical TypeScript features at Angular Toronto, that covers a lot of the NgRx typings and how they work.
Conclusion link
Most of the developers are excited about the new creator functions, and so am I. From my experience, the majority is happy that they can save keystrokes while writing NgRx code but I believe the power lies in the type-safety it provides out of the box.
Because TypeScript is growing, many libraries can benefit from the added features in each release. NgRx is one of them, without TypeScript, we wouldn't be able to create these features.
Another benefit that the creator functions bring to the table is the ability to create higher order functions, we already saw how this looked for a reducer but we can do the same thing for actions and Effects. We can even plug in our own flavors, for example, the mutableOn
reducer function that wraps the reducer with the Immer produce
function.
Throughout this article I might have sounded like a broken record, but the power that createAction
action provides is huge. The added type
property plays a huge part of it, because it can be accessed (without having to invoke the action creator function) in the reducers and effects to filter actions. This all, while staying (and making it easier) to remain type-safe. Without it, the other creator functions would not exist.
Feel free to update this blog post on GitHub, thanks in advance!
Join My Newsletter (WIP)
Join my weekly newsletter to receive my latest blog posts and bits, directly in your inbox.
Support me
I appreciate it if you would support me if have you enjoyed this post and found it useful, thank you in advance.